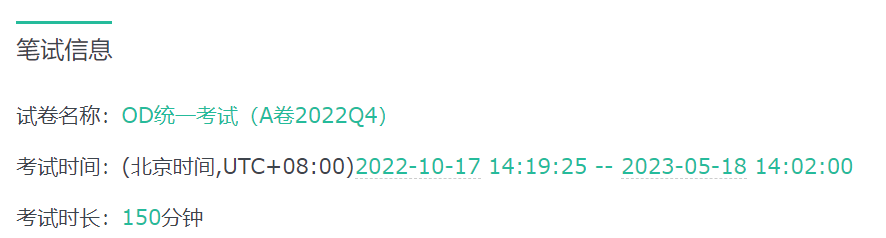
1.单入口空闲区域
/*
Author:Sanro
Time:2023.05.17 18:27:49
Blog:https://sanlangcode.com
Email:sanlangcode@163.com
Desc:
*/
import java.util.*;
import java.io.*;
import java.util.Scanner;
public class HJ202301 {
private static int startI;
private static int startJ;
public static int dfs(char[][] map, boolean[][] visited, int i, int j) {
if (i < 0 || j < 0 || i >= map.length || j >= map[0].length || map[i][j] == 'X' || visited[i][j]) {
return 0;
}
if ((i == 0 || j == 0 || i == map.length - 1 || j == map[0].length - 1) && map[i][j] == 'O' && !visited[i][j] && (i != startI || j != startJ)) {
return -10000;
}
visited[i][j] = true;
return 1 + dfs(map, visited, i - 1, j) + dfs(map, visited, i + 1, j) + dfs(map, visited, i, j - 1) + dfs(map, visited, i, j + 1);
}
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
int m = in.nextInt();
int n = in.nextInt();
char[][] inputArr = new char[m][n];
in.nextLine();
for (int i = 0; i < m; ++i) {
for (int j = 0; j < n; ++j) {
inputArr[i][j] = in.next().charAt(0);
}
}
int x = 0, y = 0;
int max = Integer.MIN_VALUE;
boolean flag = false;
for (int i = 0; i < m; ++i) {
if (inputArr[i][0] == 'O') {
boolean[][] visited = new boolean[m][n];
startI = i;
startJ = 0;
int area = dfs(inputArr, visited, i, 0);
if (area > 0 && area > max) {
flag = false;
max = area;
x = i;
y = 0;
} else if (area > 0 && area == max) {
flag = true;
}
}
if (inputArr[i][n - 1] == 'O') {
boolean[][] visited = new boolean[m][n];
startI = i;
startJ = n - 1;
int area = dfs(inputArr, visited, i, n - 1);
if (area > 0 && area > max) {
flag = false;
max = area;
x = i;
y = n - 1;
} else if (area > 0 && area == max) {
flag = true;
}
}
}
for (int j = 1; j < n - 1; ++j) {
if (inputArr[0][j] == 'O') {
boolean[][] visited = new boolean[m][n];
startI = 0;
startJ = j;
int area = dfs(inputArr, visited, 0, j);
if (area > 0 && area > max) {
flag = false;
max = area;
x = 0;
y = j;
} else if (area > 0 && area == max) {
flag = true;
}
}
if (inputArr[m - 1][j] == 'O') {
boolean[][] visited = new boolean[m][n];
startI = m - 1;
startJ = j;
int area = dfs(inputArr, visited, m - 1, j);
if (area > 0 && area > max) {
flag = false;
max = area;
x = m - 1;
y = j;
} else if (area > 0 && area == max) {
flag = true;
}
}
}
if (max <= 0) {
System.out.print("NULL");
} else if (!flag) {
System.out.print(x + " " + y + " " + max);
} else {
System.out.print(max);
}
}
}
2.汇率转换
/*
Author:Sanro
Time:2023.05.17 18:31:20
Blog:https://sanlangcode.com
Email:sanlangcode@163.com
Desc:
*/
import java.util.*;
import java.io.*;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class HJ202302 {
public static void main(String[] args) {
try(Scanner sc = new Scanner(System.in)){
int n= sc.nextInt();
sc.nextLine();
String[] Str = new String[n];
for (int i = 0; i < n; i++) {
Str[i]=sc.nextLine();
}
int res = Exchange(Str);
System.out.println(res);
}}
private static final Pattern pN = Pattern.compile("[0-9]+");
private static final Pattern pM = Pattern.compile("([a-z]|[A-Z])+");
public static int Exchange(String[] Str){
Map<String,Double> ex= new HashMap<>();
ex.put("CNY",0.01);
ex.put("fen",1.);
ex.put("JPY",.1825);
ex.put("sen",18.25);
ex.put("HKD",0.0123);
ex.put("cents",1.23);
ex.put("EUR",0.0014);
ex.put("eurocents",0.14);
ex.put("GBP",0.0012);
ex.put("pence",0.12);
double res =0. ;
for (String s : Str){
String str = s;
String num="";
String word = "";
while (str.length() > 0){
Matcher maN = pN.matcher(str);
if (maN.find()){
num =maN.group();
str = str.substring(num.length());
}
Matcher maM = pM.matcher(str);
if (maM.find()){
word =maM.group();
str = str.substring(word.length());
}
res+=Double.parseDouble(num)/ex.get(word);
}
}
return (int)res;
}
}
3.机器人活动区域
/*
Author:Sanro
Time:2023.05.17 18:31:32
Blog:https://sanlangcode.com
Email:sanlangcode@163.com
Desc:
*/
import java.util.*;
import java.io.*;
public class HJ202303 {
public static int RobotDFS(int[][] nums, int i, int j, int preNum) {
if (i < 0 || i >= nums.length || j < 0 || j >= nums[0].length) {return 0; }
int curNum = nums[i][j];
if (curNum == -1) { return 0;}
if (Math.abs(curNum - preNum) > 1) {return 0;}
nums[i][j] = -1;
int count = 1;
count += RobotDFS(nums, i - 1, j, curNum);
count += RobotDFS(nums, i + 1, j, curNum);
count += RobotDFS(nums, i, j - 1, curNum);
count += RobotDFS(nums, i, j + 1, curNum);
return count;
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String numStr = sc.nextLine();
String[] strings = numStr.split(" ");
int m = Integer.parseInt(strings[0]);
int n = Integer.parseInt(strings[1]);
int[][] nums = new int[m][n];
for (int i = 0; i < m; i++) {
String line = sc.nextLine();
String[] split = line.split(" ");
for (int j = 0; j < split.length; j++) {
nums[i][j] = Integer.parseInt(split[j]);
}
}
int Resultmax = 0;
for (int i = 0; i < m; i++) {
for (int j = 0; j < n; j++) {
int currNum = nums[i][j];
if (currNum != -1) {
Resultmax = Math.max(Resultmax, RobotDFS(nums, i, j, currNum));
}
}
}
System.out.println(Resultmax);
}
}